Powertoys is “a set of utilities for power users” that Microsoft keeps separate from Windows so they can get away with less testing. My favorite one is “Text Extractor”, which lets you OCR capture anything on the screen. Win-Shift-T:
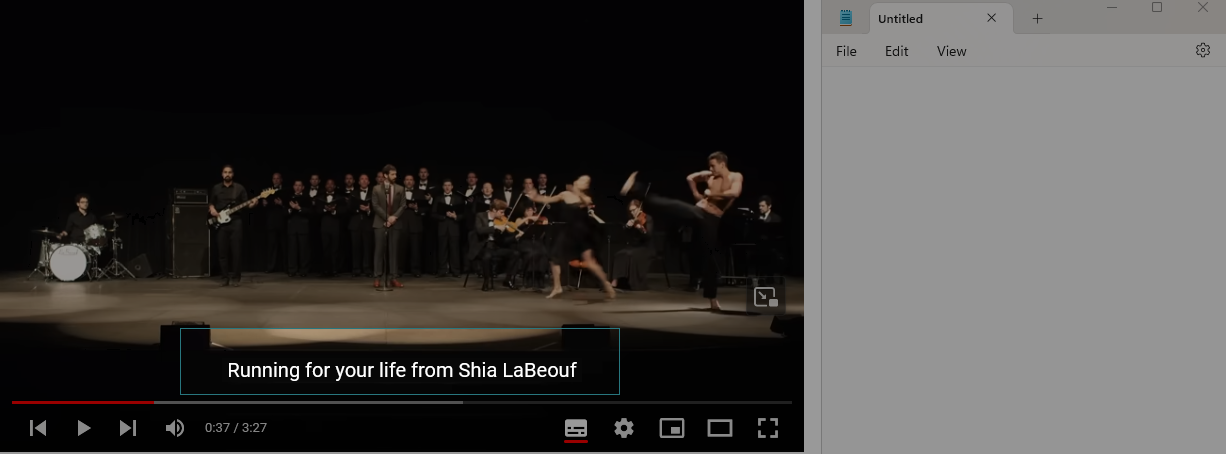
Text in the selected field is stored to your clipboard.
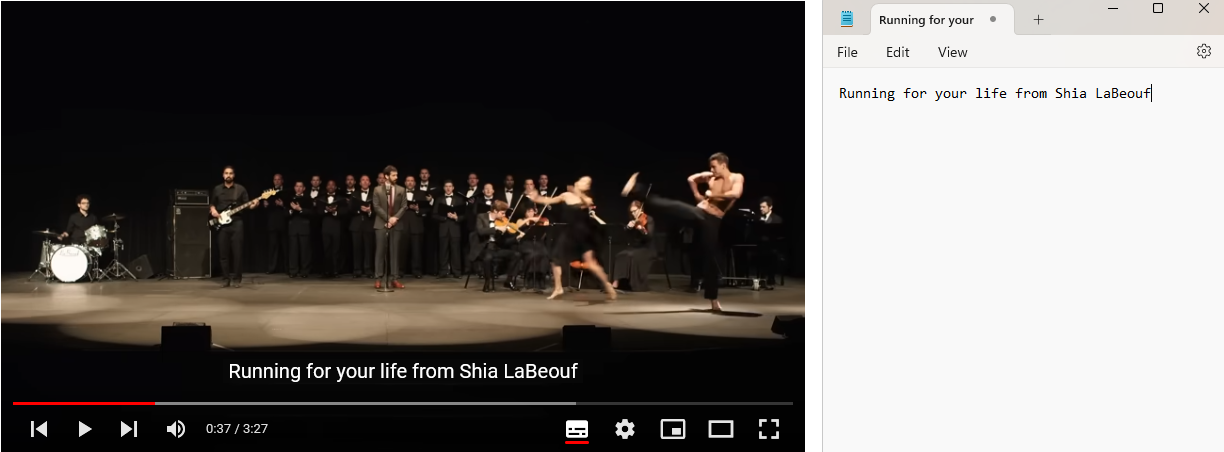
It doesn’t work well with photographs or non-computer fonts, though.
For my StrangeLoop workshop I had to do a lot of powerpoint work. To streamline this, I made over 20 AutoHotKey shortcuts to run various ribbon commands. To avoid polluting my keyboard I built them all into a general-purpose system. All shortcuts started by clicking the thumb mouse button and then quickly pressing 1-2 keys in sequence.
#IfWinActive ahk_exe POWERPNT.EXE
pp_func()
{
pp_cmd := Map("a2", {cmd: "{alt}adu.2", info: "Set animation time to .2 seconds"}
, "fh", {cmd: "{alt}hgoh", info: "Flip horizontal"} ; etc etc
)
pp_input := "?"
pp_info := ""
for pkey, pval in pp_cmd {
pp_input .= "," . pkey
pp_info .= Format("{1:-20}{2:-1}`n",pkey,pval.info)
}
ih := InputHook("L2 C T1",, pp_input)
ih.Start()
if (ih.Wait() == "Match") {
if (ih.Match == "?") {
MsgBox(pp_info)
}
else {
Send(pp_cmd[ih.Match].cmd)
}
}
}
; Mouse thumb button, you can change this to something else
XButton1::pp_func()
#IfWinActive
Pressing ?
will show all of the commands along with descriptions.
How it Works
A small helper I use to debug some python programs:
from contextlib import contextmanager
@contextmanager
def debug_error():
try:
yield
except Exception as e:
breakpoint()
quit()
### usage
with debug_error():
x = 1
y = 0
x/y # x and y will be avail in pdb
You can also put things before the yield
(which will run before the with
block), and after it (to run at the end of the block.) Anything put after it will run at the end of the block. See @contextmanager for more info.
Notes
Python 3.4 added path objects, but most people still don’t know about them. They simplify a lot of file operations:
### Without pathlib
path = "path/to/file"
# parent dir
os.path.dirname(path)
# file suffix
os.path.splitext(path)[1]
# read file
with open(path) as f:
x = f.read()
### With pathlib
path = pathlib.Path("path/to/file")
# parent dir
path.parent
# file suffix
path.suffix
# read file
x = path.read_text()
Paths are also OS-independent, so the same code will generally work on both Windows and Unix.
This one’s in Lua because I use Neovim, but it should be directly translatable to VimScript.
I often need to rerun the same commands in a specific buffer. For example, I might regularly run one command to expand an XML metafile, and then another to run one of the expanded outputs.
function LoadLocal(local_cmd)
vim.b.local_cmd = local_cmd
end
function RunLocal()
vim.cmd(vim.b.local_cmd)
end
vim.cmd [[command! -nargs=1 LoadLocal call v:lua.LoadLocal(<f-args>)]]
vim.keymap.set('n', 'gxl', RunLocal, {silent = true})
Use it like this:
Then, for that buffer and only that buffer, typing gxl
will call python on the file.
Notes
I have a few different map leaders. The spacebar is my default <leader>
for most mappings, but I also use \
for some navigational commands, gx
for triggering scripts, and ;
for insert-mode commands, like